I made a very simple homemade MIDI keyboard USB device using the Raspberry Pi Pico:
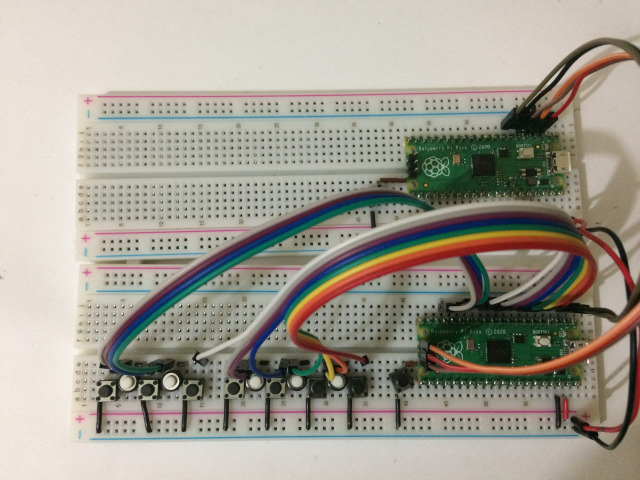
The keyboard is just 12 buttons arranged as a vague semblance of a piano keyboard (just one octave). Each button closes the connection to ground when pressed, and is connected to an input pin of the Pico which is pulled up, so when the button is pressed the input goes low.
I used TinyUSB to make the Pico act as a MIDI USB device when connected to a PC. The program is really simple: I just changed a MIDI example included in the TinyUSB project, adapting it to send MIDI notes when the buttons are pressed, with some very rudimentary debouncing for the buttons.
This project gave me an excuse to learn how to program and debug a
Pico using another Pico as the hardware debugger, using picoprobe (I
had to do it because I usually printf
debug using the USB serial,
but that obviously wouldn't work in this project). To use picoprobe
you just have to flash the Pico that will be used as the debugger with
the picoprobe firmware (picoprobe.uf2
) and make some connections
from its GPIO pins to the Pico being debugged (SWD pins and the UART
RX/TX).
Everything went really smoothly, so there's nothing much to say, other than Picoprobe is a great way to upload programs to the Pico: there's no need to reset with the Bootsel button pressed. I might start using it even when I'm not using the USB for anything else. You can also actually run GDB to debug the program live on the Pico, but I rarely use GDB like that.
On the MIDI device side of things, it works really great on Windows. I was even able to record a crappy song I played on the crappy keyboard using MidiEditor, even though there's some noticeable input lag.
On Linux, I see no input lag when dumping the MIDI events with
aseqdump
(an Alsa command line program), but I had no luck recording
input from the MIDI device using MIDI editors or other GUI programs.
Every GUI program I tried wasn't able to receive MIDI input -- most of
them just crash when trying to connect to Jack (which is installed and
running, but for some reason doesn't work), and the ones that can be
configured to use Alsa simply don't work when told to do so. I have
no energy to try to make this work, so I'll have to use Windows if I
want to do more with this project.
In any case, this was a fun little project to learn how to make the Pico into a USB device and use Picoprobe.