More progress in porting Loser Corps to the ESP32 (see all posts about the port).
The game now runs with networking enabled while using the full 320x240 resolution:
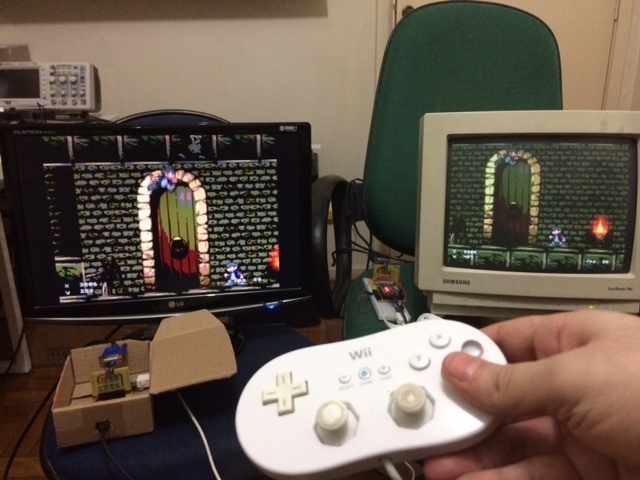
To fit WiFi and the two 320x240 framebuffers in memory, I had to make some changes: first, don't use Bluetooth -- so the Wiimote is out. Luckily I now can use the Wii Classic Controller (as shown in the picture) or Wii Nunchuk, so that's not a big deal.
Second, I had to decrease the number of transmit and receive buffers
used by the WiFi library. This can't be changed in with the Arduino
Core for the ESP32, so I can't use the Arduino IDE for this. Using
the ESP-IDF framework, this (and a lot more) can be changed with
idf.py menuconfig
:
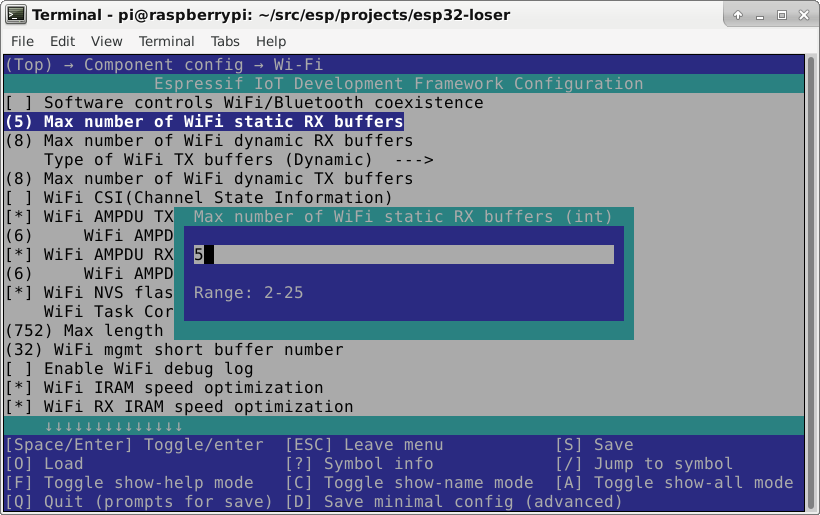
Thankfully it's not too much of a hassle to use the same code with the two APIs (which is not that surprising, since the Arduino Core is just an added layer on top of the ESP-IDF). The largest hurdle was to learn how to set the CPU clock using the ESP-IDF (it's a lot more involved than I thought, I ended up lifting code from the Arduino Core) and to support the Wiimote library, which uses the Bluetooth API from the Arduino Core (so once more I lifted some code from there).
In the end, it was fun and I learned a little bit about FreeRTOS (which the Arduino Core completely hides) in order to run my code on one of the CPUs of the ESP32 and let the WiFi and other stuff use the other one.
The other change in the game since the last update post was the addition of Wii Nunchuk and Classic Controller support via I2C with my esp32-wii-nunchuk library.
After plugging the library to the game code, I noticed that sometimes the I2C read from the controller gets stuck for a little bit. Usually that's not a big problem, but for this game any hiccup is really noticeable and annoying (the engine really wants to run at 60fps). So I had to add some functions to the library to run a loop that reads the controller state in a different CPU core than the game engine, and it ended up working really well.
The source code for the version of the game that uses the Arduino API is here: https://github.com/moefh/esp32-loser. I haven't published the version using the ESP-IDF yet, but it just adds some stuff to compile under ESP-IDF.